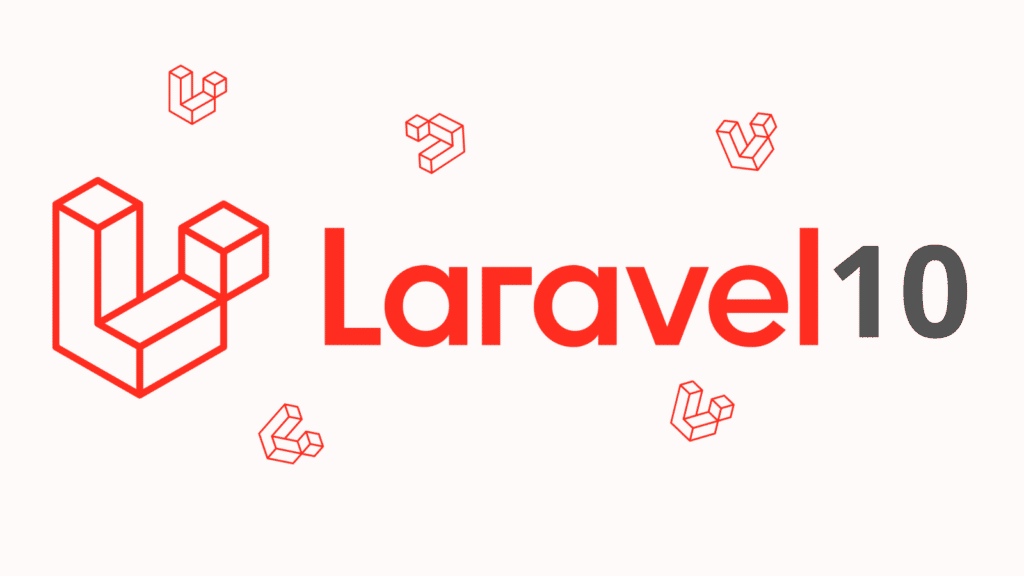
In Laravel, Eloquent provides a powerful and expressive way to define and work with database relationships. You can use various types of relationships to establish connections between your models.
Step 1 :Create Migrations:
posts table migration:
<?php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
return new class extends Migration
{
/**
* Run the migrations.
*
* @return void
*/
public function up(): void
{
Schema::create('posts', function (Blueprint $table) {
$table->id();
$table->string("name");
$table->timestamps();
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down(): void
{
Schema::dropIfExists('posts');
}
};
comments table migration:
<?php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
return new class extends Migration
{
/**
* Run the migrations.
*
* @return void
*/
public function up(): void
{
Schema::create('comments', function (Blueprint $table) {
$table->id();
$table->foreignId('post_id')->constrained('posts');
$table->string("comment");
$table->timestamps();
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down(): void
{
Schema::dropIfExists('comments');
}
};
Step 2:Create Models:
app/Models/Post.php
<?php
namespace App\Models;
use Illuminate\Database\Eloquent\Factories\HasFactory;
use Illuminate\Database\Eloquent\Model;
use Illuminate\Database\Eloquent\Relations\HasMany;
class Post extends Model
{
use HasFactory;
/**
* Get the comments for the blog post.
*
* Syntax: return $this->hasMany(Comment::class, 'foreign_key', 'local_key');
*
* Example: return $this->hasMany(Comment::class, 'post_id', 'id');
*
*/
public function comments(): HasMany
{
return $this->hasMany(Comment::class);
}
}
app/Models/Comment.php
<?php
namespace App\Models;
use Illuminate\Database\Eloquent\Factories\HasFactory;
use Illuminate\Database\Eloquent\Model;
use Illuminate\Database\Eloquent\Relations\BelongsTo;
class Comment extends Model
{
use HasFactory;
/**
* Get the post that owns the comment.
*
* Syntax: return $this->belongsTo(Post::class, 'foreign_key', 'owner_key');
*
* Example: return $this->belongsTo(Post::class, 'post_id', 'id');
*
*/
public function post(): BelongsTo
{
return $this->belongsTo(Post::class);
}
}
Step 3: Retrieve Records
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use App\Models\Post;
class PostController extends Controller
{
/**
* Write code on Method
*
* @return response()
*/
public function index(Request $request)
{
$comments = Post::find(1)->comments;
dd($comments);
}
}
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use App\Models\Comment;
class PostController extends Controller
{
/**
* Write code on Method
*
* @return response()
*/
public function index(Request $request)
{
$post = Comment::find(1)->post;
dd($post);
}
}
Step 4: Create Records
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use App\Models\Post;
use App\Models\Comment;
class PostController extends Controller
{
/**
* Write code on Method
*
* @return response()
*/
public function index(Request $request)
{
$post = Post::find(1);
$comment = new Comment;
$comment->comment = "Hi webthestuff.com";
$post = $post->comments()->save($comment);
}
}
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use App\Models\Post;
use App\Models\Comment;
class PostController extends Controller
{
/**
* Write code on Method
*
* @return response()
*/
public function index(Request $request)
{
$post = Post::find(1);
$comment1 = new Comment;
$comment1->comment = "Hi webthestuff.com Comment 1";
$comment2 = new Comment;
$comment2->comment = "Hi webthestuff.com Comment 2";
$post = $post->comments()->saveMany([$comment1, $comment2]);
}
}
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use App\Models\Post;
use App\Models\Comment;
class PostController extends Controller
{
/**
* Write code on Method
*
* @return response()
*/
public function index(Request $request)
{
$comment = Comment::find(1);
$post = Post::find(2);
$comment->post()->associate($post)->save();
}
}