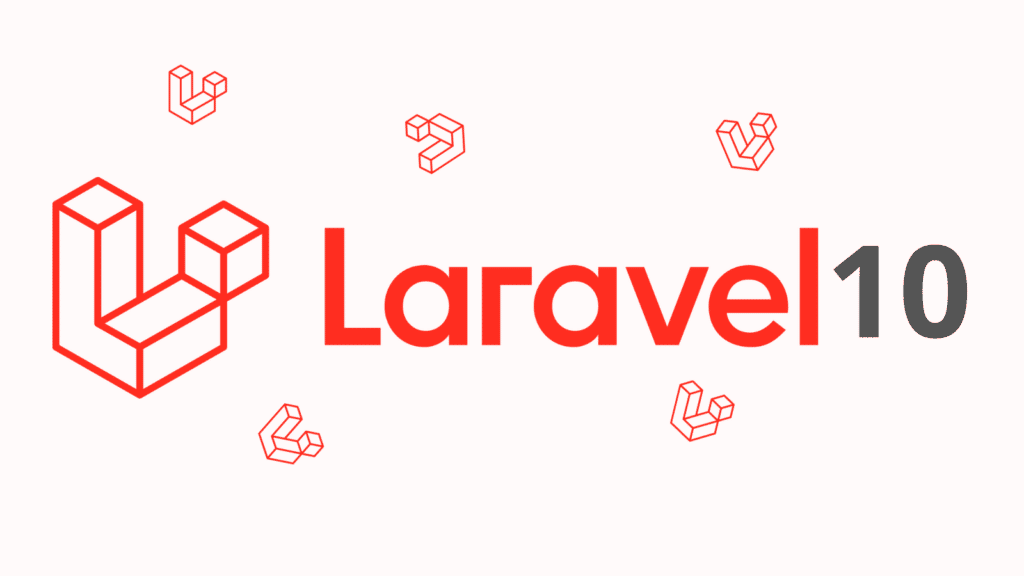
Laravel 10, Sending emails is a common requirement in web applications, and Laravel provides a powerful and convenient way to handle email sending through its built-in Mail system.
Step 1: Install Laravel App
composer create-project laravel/laravel example-emailSend
Step 2: Make Configuration
.env
MAIL_MAILER=smtp
MAIL_HOST=smtp.gmail.com
MAIL_PORT=465
MAIL_USERNAME=mygoogle@gmail.com
MAIL_PASSWORD=rrnnucvnqlbsl
MAIL_ENCRYPTION=tls
MAIL_FROM_ADDRESS=mygoogle@gmail.com
MAIL_FROM_NAME="${APP_NAME}"
Make sure you have to enable google security setting form your gmail. go to Google account and click on ‘Account’. Once you are on the ‘Account’ page, click on ‘Security’. Scroll down to the bottom and you will find ‘Less secure app access’ settings. Set as ON.
Step 3: Create Mail Class
php artisan make:mail DemoMail
app/Mail/DemoMail.php
<?php
namespace App\Mail;
use Illuminate\Bus\Queueable;
use Illuminate\Contracts\Queue\ShouldQueue;
use Illuminate\Mail\Mailable;
use Illuminate\Mail\Mailables\Content;
use Illuminate\Mail\Mailables\Envelope;
use Illuminate\Queue\SerializesModels;
class DemoMail extends Mailable
{
use Queueable, SerializesModels;
public $mailData;
/**
* Create a new message instance.
*/
public function __construct($mailData)
{
$this->mailData = $mailData;
}
/**
* Get the message envelope.
*/
public function envelope(): Envelope
{
return new Envelope(
subject: 'Demo Mail',
);
}
/**
* Get the message content definition.
*/
public function content(): Content
{
return new Content(
view: 'emails.demoMail',
);
}
/**
* Get the attachments for the message.
*
* @return array
*/
public function attachments(): array
{
return [];
}
}
Step 4: Create Controller
php artisan make:controller MailController
app/Http/Controllers/MailController.php
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use Mail;
use App\Mail\DemoMail;
class MailController extends Controller
{
/**
* Write code on Method
*
* @return response()
*/
public function index()
{
$mailData = [
'title' => 'Mail from ItSolutionStuff.com',
'body' => 'This is for testing email using smtp.'
];
Mail::to('your_email@gmail.com')->send(new DemoMail($mailData));
dd("Email is sent successfully.");
}
}
Step 5: Create Routes
routes/web.php
<?php
use Illuminate\Support\Facades\Route;
use App\Http\Controllers\MailController;
/*
|--------------------------------------------------------------------------
| Web Routes
|--------------------------------------------------------------------------
|
| Here is where you can register web routes for your application. These
| routes are loaded by the RouteServiceProvider within a group which
| contains the "web" middleware group. Now create something great!
|
*/
Route::get('send-mail', [MailController::class, 'index']);
Step 6: Create Blade File
resources/views/emails/demoMail.blade.php
<!DOCTYPE html>
<html>
<head>
<title>webthestuff.com</title>
</head>
<body>
<h1>{{ $mailData['title'] }}</h1>
<p>{{ $mailData['body'] }}</p>
<p>spsum ddssitfsfd ametdsf dipisicing elit, sed do eiusmod
tempor incididundfsft ut labore et dolore magna aliqua. Ut enim ad minim veniam,
qusds d exercitation ullamco laboris nisi ut aliquip ex ea commodo
consequat. Duis aute irure dolor dnderit insffsd voluptate velit esse
cillum dolordsdfsulla pariatur. Exceptesfoccaecfsfdat cupidatat non
proident, sunt in culpa qui officia deserunt mollit anim id est laborum.</p>
<p>Thank you</p>
</body>
</html>
Run Laravel App:
php artisan serve
Now, Go to web browser, type the given URL and see the output:
http://localhost:8000/send-mail